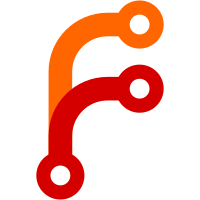
This PR includes: - Automatic build & push to docker hub when pushing to master, or when triggering a workflow dispatch on master - Automatic docker build on pull requests to validate changes - Updated the docker-compose.yaml to use the container images, rather than building locally - Added support for defining backend settings using the container environment -> All options defined in config.toml can now be loaded instead from the environment -> Order of precedence is environment definition, config.toml, and finally default inline configuration defined in config.ts - Added support for defining frontend settings using the container environment -> Added a dynamic api route to load the container environment definitions on the server, and provide them to the client -> Added a library function to fetch from the newly created API, caching the response in the session storage -> Modified existing calls to `process.env` to use the new library function -> Left the initial statically compiled environment definitions in place as a backup definition, if no environment definitions are provided Remaining tasks todo before able to merge to [ItzCrazyKns/Perplexica](https://github.com/ItzCrazyKns/Perplexica): - Add secret definitions for `DOCKER_USERNAME` and `DOCKER_PASSWORD` to [ItzCrazyKns/Perplexica](https://github.com/ItzCrazyKns/Perplexica) to ensure push to dockerhub works on base branch - Update documentation with information about changes
29 lines
661 B
TypeScript
29 lines
661 B
TypeScript
async function fetchConfig() {
|
|
try {
|
|
const response = await fetch('/api/env');
|
|
if (response.ok) {
|
|
const data = await response.json();
|
|
sessionStorage.setItem('cachedConfig', JSON.stringify(data));
|
|
return data;
|
|
} else {
|
|
throw new Error('Failed to fetch config');
|
|
}
|
|
} catch (error) {
|
|
return null;
|
|
}
|
|
}
|
|
|
|
export async function getServerEnv(envVar: string): Promise<string> {
|
|
const cachedConfig = JSON.parse(sessionStorage.getItem('cachedConfig') || 'null');
|
|
|
|
if (cachedConfig) {
|
|
return cachedConfig[envVar];
|
|
}
|
|
|
|
const data = await fetchConfig();
|
|
if (!data) {
|
|
return "";
|
|
}
|
|
|
|
return data[envVar];
|
|
}
|